RESTful API interview questions assess a candidate’s knowledge and skills in designing, developing, and testing RESTful APIs. These questions cover a wide range of topics, including RESTful API concepts, HTTP methods, status codes, and security considerations.
RESTful APIs are becoming increasingly popular due to their flexibility, scalability, and ease of use. As a result, there is a growing demand for developers who are proficient in RESTful API development. Preparing for RESTful API interview questions can help you demonstrate your skills and knowledge to potential employers.
In this article, we will explore some of the most common RESTful API interview questions. We will also provide tips on how to answer these questions and prepare for your interview.
RESTful API Interview Questions
RESTful API interview questions cover a wide range of topics, including:
- RESTful API concepts
- HTTP methods
- Status codes
- Security considerations
- Testing RESTful APIs
- RESTful API design patterns
- RESTful API best practices
- Troubleshooting RESTful APIs
These questions are designed to assess a candidate’s knowledge and skills in designing, developing, and testing RESTful APIs. By preparing for these questions, you can demonstrate your skills and knowledge to potential employers.
Here are some examples of RESTful API interview questions:
What are the six constraints of REST? What are the different HTTP methods and when should each one be used? What are some common HTTP status codes and what do they mean? What are some security considerations when designing a RESTful API? How would you test a RESTful API? What are some RESTful API design patterns? What are some RESTful API best practices? How would you troubleshoot a RESTful API?By understanding the key aspects of RESTful API interview questions, you can prepare for your interview and increase your chances of success.
RESTful API concepts
RESTful API concepts are the foundation of RESTful API interview questions. Interviewers ask questions about RESTful API concepts to assess a candidate’s knowledge and understanding of RESTful APIs. A candidate who can clearly and concisely explain RESTful API concepts is more likely to be successful in an interview.
There are many different RESTful API concepts that may be covered in an interview, including:
- The six constraints of REST
- The different HTTP methods
- Status codes
- Request and response headers
- Resource representations
- Hypermedia
In addition to understanding the individual concepts, it is also important to be able to explain how these concepts work together to create a RESTful API. For example, a candidate should be able to explain how the six constraints of REST are used to design a RESTful API, and how HTTP methods are used to perform different operations on resources.
By understanding RESTful API concepts, candidates can prepare for RESTful API interview questions and increase their chances of success.
HTTP Methods
HTTP methods are an essential part of RESTful APIs. They are used to perform different operations on resources, such as creating, reading, updating, and deleting. A good understanding of HTTP methods is essential for RESTful API developers.
In a RESTful API interview, you may be asked questions about HTTP methods. These questions may cover a range of topics, such as:
- The different HTTP methods and their uses
- When to use each HTTP method
- The relationship between HTTP methods and RESTful API design
By understanding HTTP methods, you can design and develop RESTful APIs that are efficient, scalable, and easy to use. Here are some examples of how HTTP methods are used in RESTful APIs:
- The GET method is used to retrieve a resource.
- The POST method is used to create a new resource.
- The PUT method is used to update an existing resource.
- The DELETE method is used to delete a resource.
By understanding the connection between HTTP methods and RESTful API interview questions, you can prepare for your interview and increase your chances of success.
Status codes
Status codes are an essential part of RESTful APIs. They are used to indicate the status of a request and to provide feedback to the client. A good understanding of status codes is essential for RESTful API developers.
In a RESTful API interview, you may be asked questions about status codes. These questions may cover a range of topics, such as:
- The different status codes and their meanings
- When to use each status code
- The relationship between status codes and RESTful API design
By understanding status codes, you can design and develop RESTful APIs that are efficient, scalable, and easy to use. Here are some examples of how status codes are used in RESTful APIs:
- A 200 status code indicates that the request was successful.
- A 404 status code indicates that the requested resource was not found.
- A 500 status code indicates that there was an internal server error.
By understanding the connection between status codes and RESTful API interview questions, you can prepare for your interview and increase your chances of success.
Security considerations
Security considerations are crucial in RESTful API development, as APIs provide a gateway to an application’s data and functionality. Interviewers often assess candidates’ understanding of RESTful API security practices to ensure they can create secure and robust APIs.
-
Authentication and authorization
Interviewers may ask about methods for authenticating users and authorizing their access to specific API endpoints. Common techniques include OAuth, JWTs, and API keys.
-
Data protection
Candidates should demonstrate knowledge of techniques for protecting data in transit and at rest, such as encryption, hashing, and access control mechanisms.
-
Input validation
Interviewers may inquire about strategies for validating user input to prevent malicious attacks, such as SQL injection and cross-site scripting.
-
Error handling
Robust error handling is essential for preventing attackers from exploiting system vulnerabilities. Candidates should discuss best practices for handling errors securely and providing meaningful error messages.
By understanding security considerations and their implications for RESTful API interview questions, candidates can effectively prepare for technical discussions and showcase their proficiency in securing RESTful APIs.
Testing RESTful APIs
Testing RESTful APIs is a crucial aspect of RESTful API development, ensuring that APIs meet functional and non-functional requirements. In RESTful API interviews, candidates are often evaluated on their understanding and proficiency in testing RESTful APIs.
-
Unit Testing
Interviewers may inquire about techniques for unit testing RESTful API controllers, services, and models, such as mocking dependencies and asserting expected outputs.
-
Integration Testing
Candidates should demonstrate knowledge of strategies for integration testing RESTful APIs with external systems, such as databases and third-party services.
-
Performance Testing
Interviewers may assess candidates’ understanding of performance testing tools and techniques to evaluate the scalability and responsiveness of RESTful APIs under load.
-
Security Testing
Candidates should be familiar with security testing methods for RESTful APIs, including penetration testing and vulnerability scanning, to ensure the APIs are resistant to malicious attacks.
By understanding the significance of testing RESTful APIs and its relevance in RESTful API interview questions, candidates can effectively prepare for technical discussions and showcase their proficiency in developing robust and reliable RESTful APIs.
RESTful API design patterns
RESTful API design patterns are reusable solutions to common challenges in RESTful API development. They provide a structured approach to designing and implementing RESTful APIs, ensuring consistency, maintainability, and scalability. Understanding RESTful API design patterns is essential for RESTful API developers and is often a key focus in RESTful API interview questions.
-
Layered Architecture
The layered architecture pattern divides the API into distinct layers, such as the data access layer, business logic layer, and presentation layer. This separation of concerns promotes modularity, maintainability, and testability.
-
Repository Pattern
The repository pattern provides an abstraction layer between the API and the underlying data source. It encapsulates data access operations, simplifying the development and maintenance of data access logic.
-
Service Pattern
The service pattern defines a set of reusable business logic operations. It encapsulates complex business logic, making it easier to maintain and test.
-
Factory Pattern
The factory pattern provides a way to create objects without specifying the concrete class. This promotes loose coupling between the API and the underlying implementation.
By understanding these RESTful API design patterns and their implications for RESTful API interview questions, candidates can effectively prepare for technical discussions and showcase their proficiency in designing and developing robust and scalable RESTful APIs.
RESTful API best practices
RESTful API best practices are a set of guidelines and conventions that help developers design and implement RESTful APIs that are efficient, scalable, and maintainable. These best practices cover a wide range of topics, including API design, resource representation, error handling, and security.
A good understanding of RESTful API best practices is essential for developers who want to create high-quality RESTful APIs. In a RESTful API interview, candidates may be asked questions about RESTful API best practices. These questions may cover a range of topics, such as:
- How to design a RESTful API
- How to represent resources in a RESTful API
- How to handle errors in a RESTful API
- How to secure a RESTful API
By understanding RESTful API best practices, candidates can design and develop RESTful APIs that are efficient, scalable, and maintainable. This can lead to a number of benefits, including:
- Improved performance
- Increased scalability
- Reduced maintenance costs
- Improved security
In conclusion, RESTful API best practices are an essential part of RESTful API development. By understanding and following these best practices, developers can create high-quality RESTful APIs that meet the needs of their users.
Troubleshooting RESTful APIs
Troubleshooting RESTful APIs is an essential skill for any developer who works with these APIs. RESTful APIs can be complex, and there are many things that can go wrong. Being able to troubleshoot these problems quickly and efficiently is essential for keeping your APIs up and running.
There are a number of different tools and techniques that can be used to troubleshoot RESTful APIs. Some of the most common include:
- Logging and debugging
- Unit testing
- Integration testing
- Performance testing
- Security testing
By understanding the connection between “Troubleshooting RESTful APIs” and “restful api interview questions,” candidates can effectively prepare for technical discussions and showcase their proficiency in developing robust and reliable RESTful APIs.
FAQs on RESTful API Interview Questions
Interviews for RESTful API positions often involve a range of questions to assess a candidate’s knowledge and skills in designing, developing, and troubleshooting RESTful APIs. To help you prepare effectively, we’ve compiled answers to frequently asked questions regarding RESTful API interview questions:
Question 1: What are the essential RESTful API concepts I should be familiar with?
RESTful API concepts form the foundation of RESTful API development. You should have a clear understanding of REST constraints, HTTP methods, status codes, resource representations, and hypermedia.
Question 2: Can you explain the different HTTP methods and their appropriate usage?
HTTP methods are fundamental to RESTful APIs. Understanding the functions and appropriate usage of GET, POST, PUT, DELETE, and other HTTP methods is crucial.
Question 3: How do you handle error scenarios and provide meaningful error responses in RESTful APIs?
Error handling is vital in RESTful API development. You should be able to discuss strategies for returning clear and informative error messages, along with appropriate HTTP status codes.
Question 4: What security considerations should be taken into account when designing RESTful APIs?
Security is paramount in RESTful API development. You should be familiar with authentication and authorization mechanisms, as well as techniques for preventing common attacks like SQL injection and cross-site scripting.
Question 5: How do you approach testing RESTful APIs to ensure their reliability and performance?
Testing is crucial for RESTful API development. You should be familiar with unit testing, integration testing, performance testing, and security testing techniques.
Question 6: What are some best practices for designing and developing RESTful APIs?
Adhering to RESTful API best practices ensures efficient and maintainable APIs. These practices include resource naming conventions, versioning, pagination, caching, and documentation.
By understanding and preparing for these common RESTful API interview questions, you can demonstrate your knowledge and skills, and increase your chances of success in your interview.
For further exploration, you may refer to our comprehensive article on “RESTful API Interview Questions.” Additionally, practicing with mock interviews and solving coding challenges can enhance your preparation.
Tips on Answering RESTful API Interview Questions
To excel in RESTful API interview questions, consider the following tips:
Tip 1: Understand RESTful API Concepts Thoroughly
Grasp the six constraints of REST, HTTP methods, status codes, resource representations, and hypermedia to demonstrate a solid foundation in RESTful API principles.
Tip 2: Master HTTP Methods and Their Usage
Become proficient in using GET, POST, PUT, DELETE, and other HTTP methods. Understand when to employ each method appropriately.
Tip 3: Handle Errors Gracefully
Discuss strategies for managing error scenarios effectively. Explain how to return clear error messages with suitable HTTP status codes.
Tip 4: Prioritize API Security
Emphasize your knowledge of authentication and authorization mechanisms. Describe techniques to prevent security vulnerabilities like SQL injection and cross-site scripting.
Tip 5: Apply Testing Best Practices
Highlight your understanding of unit testing, integration testing, performance testing, and security testing techniques for RESTful APIs.
Tip 6: Adhere to RESTful API Best Practices
Demonstrate familiarity with resource naming conventions, versioning, pagination, caching, and documentation practices to showcase your commitment to developing efficient and maintainable APIs.
By incorporating these tips into your preparation, you can confidently tackle RESTful API interview questions and showcase your expertise in this domain.
Conclusion
In conclusion, RESTful API interview questions assess a candidate’s knowledge and skills in designing, developing, and troubleshooting RESTful APIs. These questions cover a range of topics, including RESTful API concepts, HTTP methods, status codes, security considerations, testing, design patterns, and best practices.
Preparing for RESTful API interview questions requires a thorough understanding of these topics. Candidates should be able to explain RESTful API concepts clearly and concisely, demonstrate proficiency in using HTTP methods, and discuss strategies for handling errors, ensuring security, and applying testing best practices. Additionally, knowledge of RESTful API design patterns and adherence to best practices are essential for developing efficient and maintainable APIs.
Youtube Video:
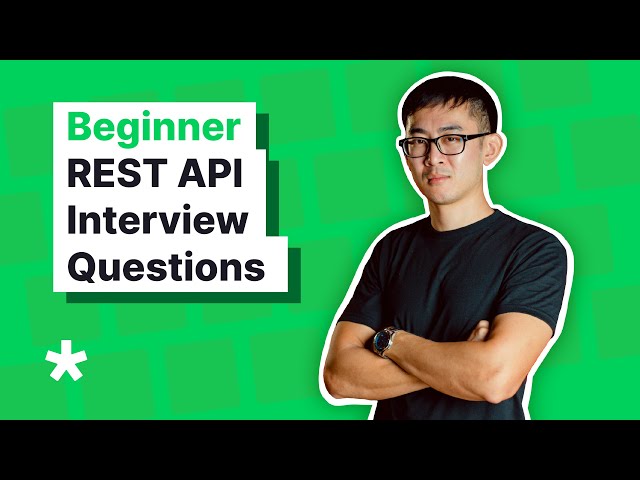